The author did not study ASP.NET, but went directly to learn ASP.NET Core. After finishing the basics of ASP.NET Core MVC, I began to learn about the operating principles of ASP.NET Core. I discovered that there is a very important "conductor" called HttpContext.
I'll quickly jot down some notes first.
Table of Contents
Preparation for Operating HttpContext
Properties and Methods of HttpContext
Practice and Testing of HttpContext Object
“Conductor” HttpContext
To understand what HttpContext does, first, look at the images.
Figure 1: Internal Network Access Program
Figure 2: Reverse Proxy Access Program
In ASP.NET Core programs, Kestrel is a cross-platform ASP.NET Core web server based on libuv. It’s okay if you don’t know about Kestrel, you can learn about it gradually later.
We can understand that external access to our program is done via Http or Https, for example, https://localhost:44337/Home/Index, which requires a URL to access a specific page.
When accessing a page, cookies, sessions, form submissions, data uploads, authentication, etc., will be created. The conductor between the external environment and the application is HttpContext.
In short, the interaction between the client and the web application is conducted through HttpContext.
Principle
ASP.NET Core is essentially a console program! The ASP.NET Core application does not listen directly to requests, but relies on the HTTP Server to forward each request to the application. The forwarded requests are equivalent to the network requests we make when browsing the web, uploading files, submitting forms, etc., which are packaged and combined into HttpContext.
It's like a customer dining in a restaurant:
- The customer needs to order food and make service requests.
- The waiter delivers the menu and requests to the kitchen.
- The chef prepares the food.
- The waiter delivers the food to the customer.
HttpContext acts as this waiter, passing information back and forth.
Preparation for Operating HttpContext
Generally speaking, we mainly focus on writing web programs without needing to worry about how the data is conducted. It’s similar to how two computers can exchange data without us needing to know whether they are using wireless WiFi, fiber optics, or copper wire cables.
When necessary, we will need to get familiar with it; enough talk, let’s start with some simple operations involving HttpContext. We will continue to analyze this object later.
If you do not need to practice, you can skip this section directly.
- Open VS (2017)
- Create a new project
- ASP.NET Core Web Application
- Web Application (Model-View-Controller)
- Open Startup.cs and add in ConfigureServices:
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>(); //don't worry about what this does yet
- Open HomeController.cs and replace the HomeController class with the following code:
-
Open the Views/Home directory and delete all other views except for Index.cshtml.
- Change the code in Index.cshtml to:
public class HomeController : Controller { private IHttpContextAccessor _accessor; public HomeController(IHttpContextAccessor accessor) { _accessor = accessor; } [HttpGet] public IActionResult Index(int? id) { var httpcontext = _accessor.HttpContext;</span><span style="color: #0000ff;">return</span><span style="color: #000000;"> View(httpcontext); } }</span></pre>
@model Microsoft.AspNetCore.Http.HttpContext @{ Layout = null; }
At this point, the preparation is complete.
The purpose of the above code is to pass the HttpContext object to the view for direct use within the view. This way, we only need to test in the view to understand it.
Properties and Methods of HttpContext
In ASP.NET Core, the system allocates a thread for each request, and HttpContext corresponds to one thread. Therefore, its class, methods, properties, etc., are effective for the current request.
Properties
Authentication |
This is no longer used and is listed here for reference only. Used for authentication (applicable in ASP.NET), not recommended by the official documentation in ASP.NET Core. The alternative is Microsoft.AspNetCore.Authentication.AuthenticationHttpContextExtensions. |
Connection |
Gets information about the underlying connection for this request. |
Features |
Gets the collection of HTTP features provided by the server and middleware available for this request. |
Items |
Gets or sets the collection of key/value pairs that can be used to share data within the scope of this request. |
Request |
The request object. |
RequestAborted |
Notifies when the underlying connection for this request is aborted, so the request operation should be canceled. |
RequestServices |
Gets or sets the IServiceProvider collection that provides access to the service container for the request. |
Response |
The response object. |
Session |
Gets or sets the object used to manage user session data for this request. |
TraceIdentifier |
Gets or sets the unique identifier for this request in tracking logs. |
User |
Gets or sets the user for this request. |
WebSockets |
Gets an object that manages the establishment of WebSocket connections for this request. |
Objects such as Item, Session, Response, etc., are frequently used. The author will elaborate on these in practical use.
HttpContext Object Practice and Testing
Request
Used to obtain the user request object, including form submission from the browser, accessed URL, query string contained in the URL, request header, and more.
Body |
Gets or sets the RequestBody stream |
ContentLength |
Gets or sets the Content-Length header |
ContentType |
Gets or sets the Content-Type header |
Cookies |
Gets or sets the Cookies |
Form |
Gets or sets the form content |
HasFormContentType |
Checks the Content-Type header for form types. |
Headers |
Gets the request headers. |
Host |
Gets or sets the host header, which can include the port |
HttpContext |
Gets or sets the request context |
IsHttps |
Detects if the current connection is HTTPS |
Method |
Gets or sets the HTTP method |
Path |
Gets or sets the current request path, which is the URL |
PathBase |
Gets or sets the RequestPathBase, which is the preceding segment of the URL, such as https://docs.microsoft.com |
Protocol |
Gets or sets the RequestProtocol. |
Query |
A collection of the query string |
QueryString |
Gets or sets the original query string to create the query collection in Request.Query |
Scheme |
Gets or sets the HTTP request scheme |
Try it out
Open Index.Cshtml and add the following code
(For clarity, I added the table)
<table> <tr> <td>RequestBody stream</td> <td> @Model.Request.Body</td> </tr> <tr> <td>Content-Length header</td> <td>@Model.Request.ContentLength</td> </tr> <tr> <td>Content-Type header</td> <td> @Model.Request.ContentType</td> </tr> <tr> <td>Cookies </td> <td>@Model.Request.Cookies</td> </tr> <tr> <td>IsHttps</td> <td>@Model.Request.IsHttps</td> </tr> <tr> <td>Host </td> <td>@Model.Request.Host</td> </tr></table>
Run the web application, and the result is as follows
After hitting F12 in the browser, you can see the content in the console. Please check sections 1 and 3 in the figure below.
Other usage methods of Request are not elaborated here; you can simply add the properties you need to test using @Model.Request. in your views.
Recommended article about Request by others: https://www.cnblogs.com/Sea1ee/p/7240943.html
Response
Request is the request made by the client to the web, while the Response is the web's response to the client's request. The author will not translate everything here.
Using Response can directly affect the server's response, set the response content, response type (sending web pages, files, images, etc.), and view response redirection before sending it.
Response should be used in controllers. The specific usage will not be elaborated here.
。
Body |
Gets or sets the response body stream. |
ContentLength |
Gets or sets the value for the |
ContentType |
Gets or sets the value of the content type response header. |
Cookies |
Gets an object that can be used to manage the cookies for this response. |
HasStarted |
Gets a value indicating whether response headers have been sent to the client. |
Headers |
Gets the response headers. |
HttpContext |
Gets the HttpContext for this response. |
StatusCode |
Gets or sets the HTTP response code. |
Methods of Response
OnCompleted(Func<Task>) |
Adds a delegate to be invoked after the response has been sent to the client. |
OnCompleted(Func<Object,Task>, Object) |
Adds a delegate to be invoked after the response has been sent to the client. |
OnStarting(Func<Task>) |
Adds a delegate to be invoked before the response headers are sent to the client. |
OnStarting(Func<Object,Task>, Object) |
Adds a delegate to be invoked before the response headers are sent to the client. |
Redirect(String) |
Returns a temporary redirect response to the client (HTTP 302). |
Redirect(String, Boolean) |
Returns a redirect response to the client (HTTP 301 or HTTP 302). |
RegisterForDispose(IDisposable) |
Registers an object for disposal after the request has completed. |
Response Extension Methods
GetTypedHeaders(HttpResponse) | |
WriteAsync(HttpResponse, String, Encoding, CancellationToken) |
Writes the given text to the response body using the specified encoding and cancellation token. |
WriteAsync(HttpResponse, String, CancellationToken) |
Writes the given text to the response body. UTF-8 encoding will be used |
Clear(HttpResponse) | |
SendFileAsync(HttpResponse, IFileInfo, Int64, Nullable<Int64>, CancellationToken) |
Uses the SendFile extension to send the given file. |
SendFileAsync(HttpResponse, IFileInfo, CancellationToken) |
Sends the given file using the SendFile extension. |
SendFileAsync(HttpResponse, String, Int64, Nullable<Int64>, CancellationToken) |
Sends the given file using the SendFile extension. |
SendFileAsync(HttpResponse, String, CancellationToken) |
Sends the given file using the SendFile extension. |
Please refer to the second part of the image below
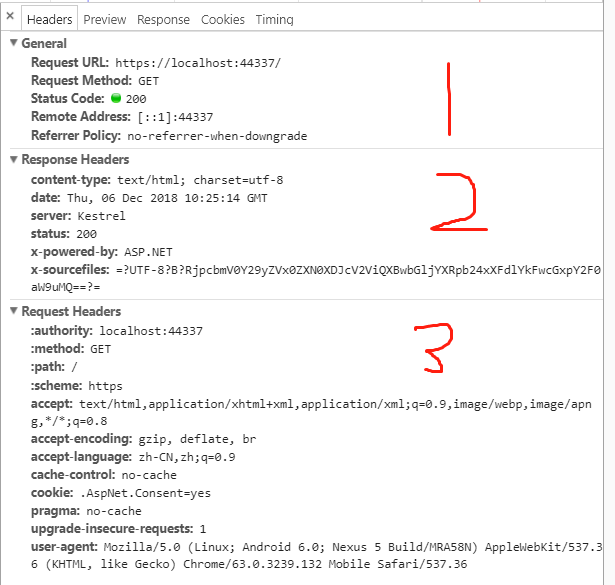
Item
If you have used ViewData, you can easily understand HttpContext.Item
HttpContext.Item is a dictionary collection of type IDictionary<TModel,TModel>. Its usage is similar to ViewData. (Don't tell me you don't know what ViewBag and ViewData are~)
Open Index.Cshtml and replace it with the following code
@model Microsoft.AspNetCore.Http.HttpContext @{ Layout = null; } @{ List<string> i = new List<string>(); i.Add("a"); i.Add("b"); i.Add("c"); i.Add("d"); i.Add("e"); i.Add("f"); i.Add("g"); i.Add("h"); i.Add("i");Model.Items[</span><span style="color: #800000;">"</span><span style="color: #800000;">Test</span><span style="color: #800000;">"</span>] =<span style="color: #000000;"> i; </span><span style="color: #008000;">/*</span><span style="color: #008000;"> Model.Items is of dictionary type Here set the key as Test with value i, its type is List<string> </span><span style="color: #008000;">*/</span>
foreach(var item in Model.Items["Test"] as List<string>) //Dictionary type, must be cast to the respective type first
{
<br> @item}
}
Result
You can use HttpContext.Item to store useful data for the current request's intention.
Other methods of HttpContext are not elaborated here. It is important to note that HttpContext is generated for a specific request.
文章评论