- 1. Determine Type
- Determine Whether Some Type
- 1.1.1 Determine Whether Type or Delegate
- 1.1.2 Determine Whether Generic
- 1.1.3 Generic Parameter Names and Constraints
- 1.1.4 Is Delegate
- 1.1.5 Access Modifiers
- 1.1.6 Sealed Classes, Static Types, Abstract Classes
- 1.1.7 Nested Class Access Levels
- 1.1.8 Attributes
- 1.1.9 Base Class, Interfaces
- 2. Type Members
- 2.1 Classes
- 2.2 Delegates
- 2.3 Interfaces
- 2.4 Nullable Types
This article mainly studies various information and identifiers of types and type members, analyzing the information through reflection operations.
The main purpose of this article is to generate output similar to the image below through reflection operations.
Before we begin, note the following:
Access modifiers in C#: public, private, protected, internal, protected internal.
Two member keywords in C#: readonly, const.
Declaration modifiers in C#: sealed, static, virtual, new, abstract, override.
Based on the reflection type objects, we can roughly categorize them as: classes, value types, arrays, structs, enums, interfaces, abstract classes, delegates, events, various generics (generic classes, generic methods, generic constructors, etc.).
This article took me one and a half days to write. Besides writing, I reviewed a lot of documentation, created numerous projects, and conducted extensive testing and validation before compiling the results.
This series is now at its ninth installment.
1. Determine Type
To analyze type information from Type
, the author has organized it using a mind map as shown in the figure.
Determine Whether Some Type
Generally speaking, if there are two Type
objects, to determine whether the types reflected by the two Type
objects are the same type, you can use ==
.
Type A = typeof(ClassA);
Type B = typeof(ClassB);
Console.WriteLine(A == B);
1.1 Classes and Delegates
1.1.1 Determine Whether Type or Delegate
The Type.IsClass
property can determine whether a type is a class or a delegate. Valid types include ordinary classes (including generics), abstract classes (abstract class), and delegates (delegate).
It can exclude value types and interfaces, such as simple value types, structs, enums, and interfaces.
1.1.2 Determine Whether Generic
The Type.IsGenericType
property can determine whether a class or delegate is of a generic type.
The Type.IsGenericTypeDefinition
property can determine whether a Type
is a generic type with unbound parameters.
The Type.IsConstructedGenericType
property determines whether a Type
can create a generic instance.
If it is a generic type with bound parameters, you can instantiate the type using methods like Activator.CreateInstance()
.
Experimental process:
Create three types
public delegate void DeA();
public delegate void DeB(T t);
public class ClassC<T>
{
public ClassC(T t) { }
}</code></pre>
Print the output
// Regular delegate
Type typeA = typeof(DeA);
Console.WriteLine("Type Name: " + typeA.Name);
Console.WriteLine("Is Class or Delegate: " + typeA.IsClass);
Console.WriteLine("Is Generic: " + typeA.IsGenericType);
Console.WriteLine("Is Generic Type Definition: " + typeA.IsGenericTypeDefinition);
Console.WriteLine("Can Create Instance with This Type: " + typeA.IsConstructedGenericType);
// Generic delegate, unbound parameter type
Type typeB = typeof(DeB<>);
Console.WriteLine("\n\nType Name: " + typeB.Name);
Console.WriteLine("Is Class or Delegate: " + typeB.IsClass);
Console.WriteLine("Is Generic: " + typeB.IsGenericType);
Console.WriteLine("Is Generic Type Definition: " + typeB.IsGenericTypeDefinition);
Console.WriteLine("Can Create Instance with This Type: " + typeB.IsConstructedGenericType);
// Generic delegate, bound parameter type
Type typeBB = typeof(DeB<int>);
Console.WriteLine("\n\nType Name: " + typeBB.Name);
Console.WriteLine("Is Class or Delegate: " + typeBB.IsClass);
Console.WriteLine("Is Generic: " + typeBB.IsGenericType);
Console.WriteLine("Is Generic Type Definition: " + typeBB.IsGenericTypeDefinition);
Console.WriteLine("Can Create Instance with This Type: " + typeBB.IsConstructedGenericType);
// Generic class, unbound parameter
Type typeC = typeof(ClassC<>);
Console.WriteLine("\n\nType Name: " + typeC.Name);
Console.WriteLine("Is Class or Delegate: " + typeC.IsClass);
Console.WriteLine("Is Generic: " + typeC.IsGenericType);
Console.WriteLine("Is Generic Type Definition: " + typeC.IsGenericTypeDefinition);
Console.WriteLine("Can Create Instance with This Type: " + typeC.IsConstructedGenericType);
// Generic type, bound parameter
Type typeD = typeof(ClassC<int>);
Console.WriteLine("\n\nType Name: " + typeD.Name);
Console.WriteLine("Is Class or Delegate: " + typeD.IsClass);
Console.WriteLine("Is Generic: " + typeD.IsGenericType);
Console.WriteLine("Is Generic Type Definition: " + typeD.IsGenericTypeDefinition);
Console.WriteLine("Can Create Instance with This Type: " + typeD.IsConstructedGenericType);</code></pre>
1.1.3 Generic Parameter Names and Constraints
Retrieve the names of generic parameters when obtaining the generic type definition.
public class MyClass { }
Type type = typeof(MyClass<,,,,>);
var types = ((System.Reflection.TypeInfo)type).GenericTypeParameters;
foreach (var item in types)
{
Console.WriteLine(item.Name);
}</code></pre>
Output
T1
T2
T3
T4
T5
TypeInfo
is used to handle various types of generic type declarations.
In the third section of the article titled C# Reflection and Attributes (Part IV): Instantiating Types, we explored various instantiation methods for generics.
Generic Constraints
For classes and methods, when using generic versions, it may involve generic constraints that we need to resolve.
In Type
, the GetGenericParameterConstraints
and GenericParameterAttributes
properties can determine constraint types.
Constraint
Description
where T : struct
Value type
where T : class
Type parameter must be a reference type. This constraint also applies to any class, interface, delegate, or array type
where T : notnull
Type parameter must be a non-nullable type
where T : unmanaged
Type parameter must be a non-nullable unmanaged type, which is similar to struct, but unmanaged is not safe.
where T : new()
Type parameter must have a public parameterless constructor. When used with other constraints, the new()
constraint must be specified last. The new()
constraint cannot be combined with struct
and unmanaged
constraints.
where T :
<Base Class Name>
Type parameter must be the specified base class or derived from the specified base class
where T :
<Interface Name>
Type parameter must be the specified interface or implement the specified interface. Multiple interface constraints may be specified. The constraint interface can also be generic.
where T : U
The type parameter provided for T must be for the parameter provided for U or derived from the parameter provided for U
GetGenericParameterConstraints
can obtain parameter types, but it is only valid for struct
, class
, <Base Class Name>, <Interface Name>, and T : U
.
- There are complex conflict relationships among these constraints, which are not detailed here.
- Some types themselves represent multiple constraints, for example,
struct
itself contains new()
and notnull
.
- Some constraint conditions can be combined.
From the above, it's clear that parsing generic constraints is not an easy task.
GenericParameterAttributes Enumeration
However, let’s categorize the combinations under different circumstances to clarify the relationship between Type
and GenericParameterAttributes
.
First, let's take a look at the GenericParameterAttributes
enumeration, which is used to describe the constraints of generic parameters on generic classes or methods.
public enum GenericParameterAttributes
{
None = 0, // No special conditions
Covariant = 1, // The generic type parameter is covariant
Contravariant = 2, // The generic type parameter is contravariant
VarianceMask = 3, // Collection of Contravariant and Covariant
ReferenceTypeConstraint = 4, // Reference type
NotNullableValueTypeConstraint = 8, // Is a value type and not nullable
DefaultConstructorConstraint = 16, // Parameterless constructor
SpecialConstraintMask = 28 // Collection of all special markers
}
Next, let’s examine the different constraint conditions and the corresponding values of GenericParameterAttributes
enumeration.
Generic Constraints Relationships
Generic constraints have various conflict relationships and constraint characteristics, let’s enumerate them through tables and images.
.
Constraint
Type
Enum Values
Conflict
Must be at the beginning
struct
Value Type
8,16
Can only be used alone
Yes
class
4
struct, notnull, unmanaged, <base class name>
Yes
notnull
0
struct, class, unmanaged
Yes
unmanaged
struct
8,16
Can only be used alone
Yes
new()
16
struct, unmanaged
Must be at the end
<base class name>
<base class name>
0
struct, notnull, unmanaged
Yes
<interface name>
<interface name>
0
struct, unmanaged
No
T : U
U
0
struct
No
Note: Using T : U
will not prompt conflicts with other constraints, but if there are conflicts in inherited constraints, errors may occur at compile time or runtime.
When writing code for <interface name>, there may not be conflicts with other constraints, but some constraints cannot be used together.
unmanaged, BaseInterFace
can be used as constraints, but unmanaged should be a non-managed type, which we will not consider here.
Generic constraint relationships are shown in the image below:
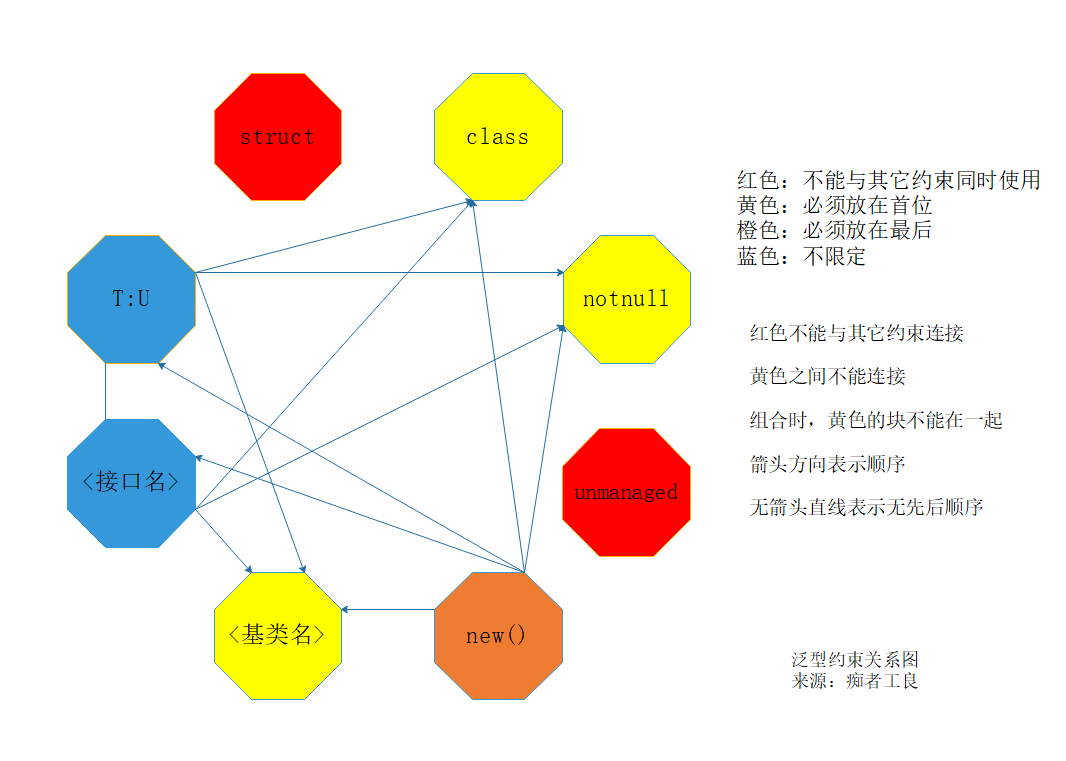
After looking at the image, do you feel that the ideas are clearer?~
There are many generic constraints, and they can be combined in various ways. However, from the above image, we can deduce the combinations as follows:
①(Red)
②(Yellow)(N blue)
③(Yellow)(N blue)(Orange)
④(Any color)
⑤(N blue)
Since there is a lot of code, it will not be displayed here. The code has been uploaded to Gitee Parsing Generics
For reflective operations on generics, please refer here https://docs.microsoft.com/zh-cn/dotnet/csharp/programming-guide/generics/generics-and-reflection
1.1.4 Is Delegate
Through previous operations, we can filter out whether a type is a type or a delegate. To determine if a type is a delegate, you can use IsSubclassOf()
which can check if a Type is a delegate type.
IsSubclassOf()
can check whether the current Type derives from the Type provided as an argument.
type.IsSubclassOf(typeof(Delegate));
Additionally, there is a multicast delegate MulticastDelegate
which can be checked using
type.IsSubclassOf(typeof(MulticastDelegate))
1.1.5 Access Modifiers
Classes and delegates declared within a namespace can only use the public and internal access modifiers. If not specified, the default is internal.
Two properties of Type IsPublic
and IsNotPublic
can be used to identify this.
Testing:
public class A { }
internal class B { }
class C { }
Output in Main:
Type typeA = typeof(A);
Type typeB = typeof(B);
Type typeC = typeof(C);
Console.WriteLine(typeA.Name);
Console.WriteLine("Is public: "+typeA.IsPublic);
Console.WriteLine("Is protected: " + typeA.IsNotPublic);
Console.WriteLine("\n"+typeB.Name);
Console.WriteLine("Is public: " + typeB.IsPublic);
Console.WriteLine("Is protected: " + typeB.IsNotPublic);
Console.WriteLine("\n" + typeC.Name);
Console.WriteLine("Is public: " + typeC.IsPublic);
Console.WriteLine("Is protected: " + typeC.IsNotPublic);</code></pre>
Output Result:
A
Is public: True
Is protected: False
B
Is public: False
Is protected: True
C
Is public: False
Is protected: True
1.1.6 Sealed Classes, Static Types, Abstract Classes
A sealed class is a type that cannot be inherited, and can be determined using the property IsSealed
of Type.
public sealed class A { }
Console.WriteLine(typeof(A).IsSealed);
Sealed can also modify delegates.
To determine if it is an abstract class, use:
public abstract class MyClass { }
Console.WriteLine(typeof(MyClass).IsAbstract);
When defining a class, static, abstract, and sealed cannot be combined together.
If a class is a static class, then both IsSealed
and IsAbstract
are true.
There are no properties or methods in Type to determine if a class is a static class, but the above methods can indicate if it is a static class.
We can conduct an experiment:
public sealed class A { }
public abstract class B { }
public static class C { }
Type typeA = typeof(A);
Type typeB = typeof(B);
Type typeC = typeof(C);
Console.WriteLine("Sealed Class:");
Console.WriteLine("IsSealed:" + typeA.IsSealed);
Console.WriteLine("IsAbstract:" + typeA.IsAbstract);
Console.WriteLine("\nAbstract Class:");
Console.WriteLine("IsSealed:" + typeB.IsSealed);
Console.WriteLine("IsAbstract:" + typeB.IsAbstract);
Console.WriteLine("\nStatic Class:");
Console.WriteLine("IsSealed:" + typeC.IsSealed);
Console.WriteLine("IsAbstract:" + typeC.IsAbstract);</code></pre>
Output Result:
Sealed Class:
IsSealed:True
IsAbstract:False
Abstract Class:
IsSealed:False
IsAbstract:True
Static Class:
IsSealed:True
IsAbstract:True
1.1.7 Nested Class Access Permissions
Below are the properties related to nested types of Type. Classes and delegates can both use these properties.
Property
Description
IsNested
Gets a value indicating whether the current Type object represents a type that is defined within the definition of another type.
IsNestedAssembly
Gets a value indicating whether the Type is nested and is only visible within its own assembly.
IsNestedFamANDAssem
Gets a value indicating whether the Type is nested and is only visible to classes that belong to the same family and the same assembly.
IsNestedFamily
Gets a value indicating whether the Type is nested and is only visible within its own family.
IsNestedFamORAssem
Gets a value indicating whether the Type is nested and is only visible to classes that belong to its own family or assembly.
IsNestedPrivate
Gets a value indicating whether the Type is nested and declared as private.
IsNestedPublic
Gets a value indicating whether the class is nested and declared as public.
1.1.8 Attributes
There are two ways to obtain attributes:
- Call the GetCustomAttributes method of Type or MemberInfo;
- Call the Attribute.GetCustomAttribute or Attribute.GetCustomAttributes methods;
In C# Reflection and Attributes (Seven): Custom Attributes and Applications, a detailed introduction to the use of attributes is provided, which will not be repeated here.
1.1.9 Base Class and Interface
Property
Description
BaseType
Gets the type from which the current Type directly inherits.
Method
Description
GetInterface(String)
Searches for an interface with the specified name.
GetInterfaces()
When overridden in a derived class, gets all interfaces implemented or inherited by the current Type.
Type type = typeof(List<>);
Console.WriteLine("The base class of List<> is: " + type.BaseType);
Console.WriteLine("Interfaces inherited by List<>:");
Type[] types = type.GetInterfaces();
foreach (var item in types)
{
Console.WriteLine(item.Name);
}</code></pre>
1.2 Value Types
Type.IsValueType
can determine whether a Type is a value type, including simple value types, structs, and enums.
Type.IsEnum
checks whether the Type is an enum.
Type.IsPrimitive
checks whether the Type is a primitive type.
The following process can determine the value type of a property of a type:
public enum MyTest
{
None = 0, // Not a value type
Enum = 1, // Enum
Struct = 2, // Struct
Base = 3 // Primitive Type
}
public static MyTest Test(Type type)
{
if (!type.IsValueType)
return MyTest.None;
if (type.IsEnum)
return MyTest.Enum;
return type.IsPrimitive ? MyTest.Base : MyTest.Struct;
}</code></pre>
For enum Type, the following methods help to retrieve enum information:
Method
Description
GetElementType()
When overridden in a derived class, returns the Type of the objects contained or referenced by the current array, pointer, or reference type.
GetEnumName(Object)
Returns the name of the constant with the specified value in the current enumeration type.
GetEnumNames()
Returns the names of the members of the current enumeration type.
GetEnumUnderlyingType()
Returns the underlying type of the current enumeration type.
GetEnumValues()
Returns an array of the values of each constant in the current enumeration type.
1.3 Interfaces
Type.IsInterface
property checks whether the Type is an interface.
1.4 Arrays
IsArray
checks if it is an array, while GetArrayRank()
retrieves the number of dimensions of the array.
GetElementType
can get the element type of an array.
IsSZArray
checks if it is a jagged array, and IsVariableBoundArray
checks for one-dimensional or multi-dimensional arrays.
IsSZArray
and IsVariableBoundArray
are available only in .NET Core 2.0 and later, and .NET Standard 2.1 and later.
Type a = typeof(int[,,,,]);
Console.WriteLine(a.Name);
Console.WriteLine("Array Element Type: " + a.GetElementType());
Console.WriteLine("Is Array: " + a.IsArray);
Console.WriteLine("Jagged Array: " + a.IsSZArray);
Console.WriteLine("One-dimensional or Multi-dimensional: " + a.IsVariableBoundArray);
Console.WriteLine("Array Dimensions: " + a.GetArrayRank());
Console.WriteLine("\n\n");
Type b = typeof(int[][][][]);
Console.WriteLine(b.Name);
Console.WriteLine("Array Element Type: " + b.GetElementType());
Console.WriteLine("Is Array: " + b.IsArray);
Console.WriteLine("Jagged Array: " + b.IsSZArray);
Console.WriteLine("One-dimensional or Multi-dimensional: " + b.IsVariableBoundArray);
Console.WriteLine("Array Dimensions: " + b.GetArrayRank());</code></pre>
However, GetElementType()
cannot obtain the initial element type at once, and GetArrayRank
is ineffective for jagged arrays.
The following methods can quickly parse the value types of jagged arrays.
。
// Can only parse value types and system fundamental types, such as int, etc.
public static (Type, int) Test(Type type)
{
if (!type.IsSZArray)
return (type, 0);
int num = 0;
Type that = type;
while (true)
{
that = that.GetElementType();
num += 1;
if (that.IsPrimitive)
break;
}
return (that, num);
}
Invocation
Type b = typeof(int[][][][]);
var result = Test(b);
Console.WriteLine("Element Type: " + result.Item1);
Console.WriteLine("Jagged Count: " + result.Item2);
For complex interleaved arrays, string processing can be used.
2. Type Members
Through the operations of the first chapter, the assembly outline can already be resolved. Now we will begin to obtain the internal details of types to construct clearer information.
The process of resolving type structures is roughly as follows
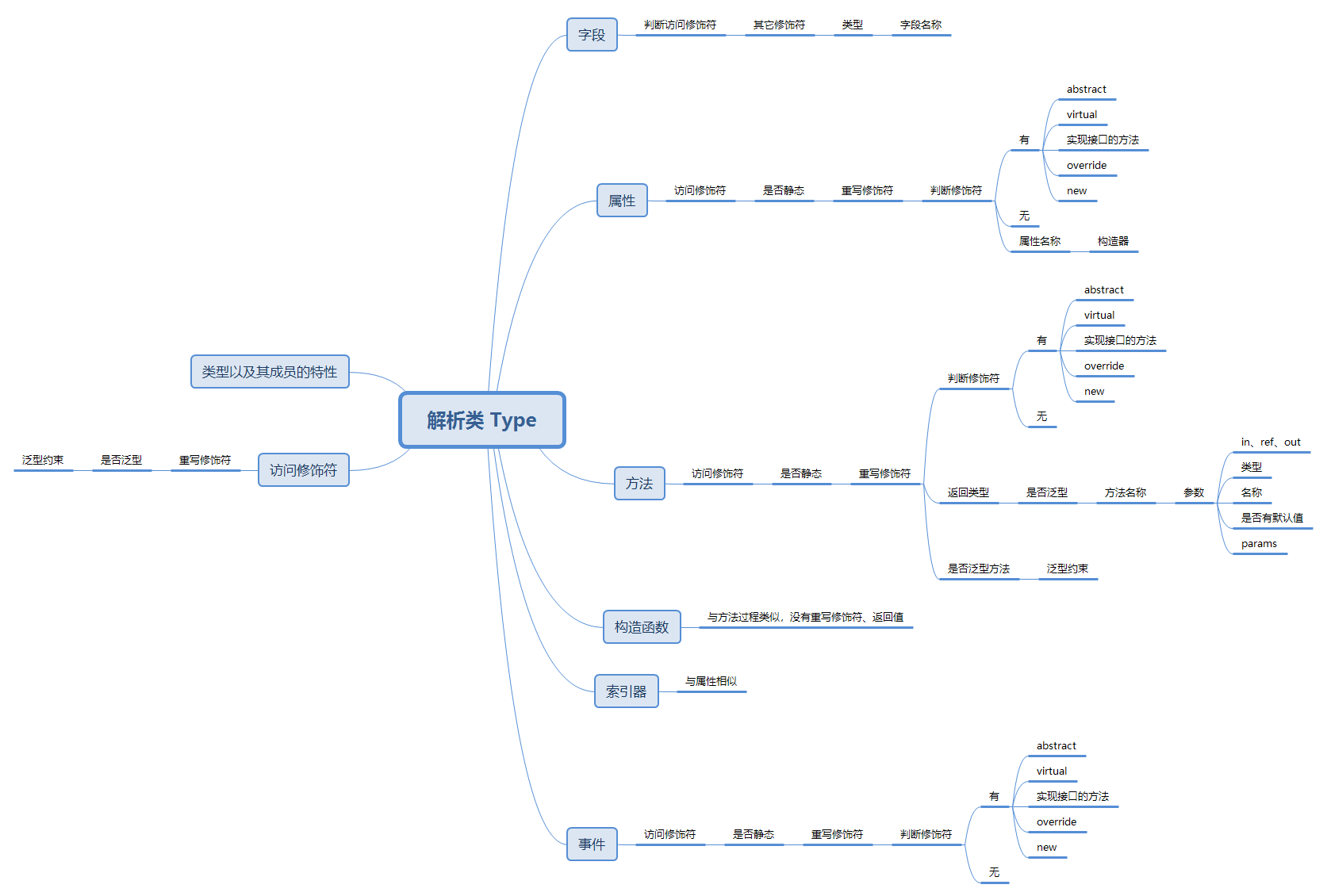
2.1 Class
A class consists of one or more of the following members:
Member Type
Description
PropertyInfo
Information about the properties of the type
FieldInfo
Information about the fields of the type
ConstructorInfo
Information about the constructors of the type
MethodInfo
Methods of the type
ParameterInfo
Parameters of the constructors or methods
EventInfo
Events of the type
As for attributes, they have already been explained in "C# Reflection and Attributes (Seven): Custom Attributes and Applications", so they will not be elaborated here.
2.1.1 Access Modifiers
The public and private modifiers are very easy to determine;
The C# keywords protected
and internal
have no significance in IL and will not be used in the reflection API. This means that from the perspective of reflection, these two access modifiers are ineffective; however, for information retrieval, it is still necessary to find a way to resolve them.
protected, internal, and protected internal have no significance for reflection invocation, but it is still necessary to find a way to resolve them for information retrieval.
To determine whether it is internal
, IsAssembly
can be used; to determine whether it is protected internal
, IsFamilyOrAssembly
can be used; if both properties return false
, then it is protected
.
Property
Description
IsAssembly
Indicates whether it is internal
IsFamily
Indicates whether it is protected
IsFamilyOrAssembly
Determines whether it is protected internal
Note: protected internal
and internal protected
are the same.
The following method can determine and return the name of the access modifier
public static string Visibility(FieldInfo field)
{
return
field.IsPublic ? "public" :
field.IsPrivate ? "private" :
field.IsAssembly ? "internal" :
field.IsFamily ? "protected" :
field.IsFamilyOrAssembly ? "protected internal" :
null;
}
2.1.2 Other Modifiers
Three modifiers: readonly, static, const; const cannot co-exist with other modifiers.
Property
Description
IsLiteral
Gets a value indicating whether this value is written at compile time and cannot be changed
IsStatic
Indicates the static fields, noting that const is also static.
IsInitOnly
Gets a value indicating whether this field can only be set in the constructor's body
The following method can determine and return the corresponding modifier
public static string Only(FieldInfo field)
{
if (field.IsLiteral)
return "const";
if (field.IsStatic && field.IsInitOnly)
return "readonly static";
if (field.IsStatic)
return "static";
if (field.IsInitOnly)
return "readonly";
return string.Empty;
}</code></pre>
const int a;
using IsStatic
returns true
, because const is also static.
2.1.3 Fields
Through sections 2.1.1 and 2.1.2, information about fields can be resolved.
Let’s test it.
Define a type
public class MyClass
{
public int a;
internal int b;
protected int c;
protected internal int d;
private int e;
public readonly static float f = 1;
}
Output parsed data
Type type = typeof(MyClass);
FieldInfo[] fields = type.GetFields(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.GetField | BindingFlags.Static | BindingFlags.Instance);
IEnumerable fields1 = type.GetRuntimeFields();
foreach (var item in fields)
{
StringBuilder builder = new StringBuilder();
builder.Append(GetVisibility(item) + " ");
builder.Append(GetRead(item) + " ");
builder.Append(item.FieldType.Name + " ");
builder.Append(item.Name + " ;");
Console.WriteLine(builder.ToString());
}
Because reflection primarily displays metadata, and properties with get;set;
will automatically generate private fields, the above code will also display those. Change the acquisition condition to BindingFlags.Public | BindingFlags.GetField | BindingFlags.Static | BindingFlags.Instance
.
2.1.4 Methods and Parameters
Excluding Property Methods
When we write a property, the compiler generates corresponding get and set methods at compile time. Generally, we only need to display methods written by programmers, not those generated by the system.
The methods generated by the system for properties will carry a System.Runtime.CompilerServices.CompilerGeneratedAttribute
attribute, which can be used to exclude system-generated methods.
public static bool IsPropertyOfAttr(MethodInfo method)
{
return method.GetCustomAttributes().Any(x => x.GetType() == typeof(System.Runtime.CompilerServices.CompilerGeneratedAttribute));
}
Method Access Modifiers
The code to determine the method access modifier is as follows
public static string GetVisibility(MethodInfo method)
{
return
method.IsPublic ? "public" :
method.IsPrivate ? "private" :
method.IsAssembly ? "internal" :
method.IsFamily ? "protected" :
method.IsFamilyOrAssembly ? "protected internal" :
null;
}
The relevant explanations have already been provided, so they will not be elaborated here.
Override and Hide Keywords
Methods can be modified by the following keywords: virtual, override, abstract, new;
In terms of inheritance relationships, one method may be virtual or abstract. After inheritance, the overridden method can be marked as override or new; abstract methods must always use override.
The following properties can distinguish modifiers:
IsAbstract
, IsVirtual
, IsHideBySig
, IsFinal
.
Methods marked with virtual, override, abstract, new all yield IsHideBySig
as true; this property can be used to determine if the method is marked with abstract, override, etc.
For methods marked with virtual or override, IsVirtual
is true; for methods marked with new, IsVirtual
is false.
However, a method that implements an interface method will return true
for both IsVirtual
and IsHideBySig
.
Thus, to distinguish between virtual
and override
, if the current method overrides a method of the parent class, using MethodInfo.GetBaseDefinition()
will return the method defined by the parent class that is being overridden; if not, it will return the method itself.
IsVirtual
can determine whether the current method is override-able.
However, a method defined in the current class, such as public string Test(){}
, can be overridden, making it easily confused with new
. Therefore, a final check is needed.
When obtaining a MethodInfo, to distinguish the above modifiers, the following code workflow can be used.
// virtual override abstract new
public static string IsOver(Type type,MethodInfo method)
{
// Absence of relevant information indicates the absence of the above keyword modifiers
if (!method.IsHideBySig)
return string.Empty;
// Whether it is an abstract method
if (method.IsAbstract)
return "abstract";
// virtual, override, and interface-implemented methods
if (method.IsVirtual)
{
// Interface-implemented methods
if (method.IsFinal)
return string.Empty;
// If not overridden, it is virtual
if (method.Equals(method.GetBaseDefinition()))
return "virtual";
else
return "override";
}
// new
else
{
// If the method is defined in the current type, just a regular method
if (type == method.DeclaringType)
return string.Empty;
return "new";
}
}</code></pre>
Getting Return Type
Information about the return type can be obtained from ReturnParameter
, ReturnType
, and ReturnTypeCustomAttributes
.
ReturnTypeCustomAttributes
is used to obtain attribute information, which will not be processed here.
// Get return type
public static string GetReturn(MethodInfo method)
{
Type returnType = method.ReturnType;
ParameterInfo returnParam = method.ReturnParameter;
if (returnType == typeof(void))
return "void";
if (returnType.IsValueType)
{
// Check if it's a return type like (type1,type2)
if (returnParam.ParameterType.IsGenericType)
{
Type[] types = returnParam.ParameterType.GetGenericArguments();
string str = "(";
for (int i = 0; i < types.Length; i++)
{
str += types[i].Name;
if (i < types.Length - 1)
str += ",";
}
str += ")";
return str;
}
return returnType.Name;
}
// Here, complex return types like arrays, generics, etc. will not be handled for now.
return returnType.Name;
}</code></pre>
method.ReturnType
and method.ReturnParameter.ParameterType
are the same.
Generally, using ReturnType
will suffice, while some special syntax may require using ReturnParameter
. The author has not yet encountered any scenarios where distinguishing becomes necessary.
Whether the Method is Asynchronous
The following code can be used to determine if the method is asynchronous
public static string GetAsync(MethodInfo info)
{
return info.GetCustomAttribute(typeof(AsyncStateMachineAttribute))==null?"":"async ";
}
Generic Methods
The following code can determine whether it is a generic method and return its name.
.
// Determine if the method is a generic method and return the generic name
public static string GetMethodName(MethodInfo method)
{
if (!method.IsGenericMethod)
return method.Name;
Type[] types = method.GetGenericArguments();
string str = method.Name + "<";
for (int i = 0; i < types.Length; i++)
{
str += types[i].Name;
if (i < types.Length - 1)
str += ",";
}
str += ">";
return str;
}
Method Parameters
Step 1: Determine if the parameter has the in, ref, out modifiers; if so, the type name will be followed by the character &
; if params, it will have a ParamArrayAttribute
attribute.
Step 2: Get the parameter type; if it has in, ref, out modifiers, the type name will be followed by an &
that needs to be removed;
Step 3: Check if there is a default value; if a default value exists, return it.
// Parse method parameters
public static string GetParams(MethodInfo method)
{
ParameterInfo[] parameters = method.GetParameters();
if (parameters.Length == 0)
return string.Empty;
int length = parameters.Length - 1;
StringBuilder str = new StringBuilder();
for (int i = 0; i <= length; i++)
{
str.Append(InRefOut(parameters[i]) + " ");
// No processing for complex types here
str.Append(GetParamType(parameters[i]) + " ");
str.Append(parameters[i].Name);
str.Append(HasValue(parameters[i]) + " ");
if (i < length)
str.Append(",");
}
return str.ToString();
}
public static string InRefOut(ParameterInfo parameter)
{
// in, ref, out, the type will have a & symbol at the end
if (parameter.ParameterType.Name.EndsWith("&"))
{
return
parameter.IsIn ? "in" :
parameter.IsOut ? "out" :
"ref";
}
if (parameter.GetCustomAttributes().Any(x => x.GetType() == typeof(ParamArrayAttribute)))
return "params";
return string.Empty;
}
// Get parameter type
public static string GetParamType(ParameterInfo parameter)
{
string typeName = parameter.ParameterType.Name;
if (typeName.EndsWith("&"))
typeName = typeName.Substring(0, typeName.Length - 1);
return typeName;
}
// Check if it is an optional parameter and if it has a default value
public static string HasValue(ParameterInfo parameter)
{
if (!parameter.IsOptional)
return string.Empty;
object value = parameter.DefaultValue;
return " = " + value.ToString();
}</code></pre>
Application of Learning
After learning how to acquire and parse method information, we can practice here.
Define the following types; what we ultimately need is MyClass.
interface A
{
void TestA();
}
public abstract class B
{
public abstract void TestB();
}
public abstract class C : B
{
public virtual void TestC()
{
}
public virtual void TestD()
{
}
}
public class MyClass : C, A
{
public void TestA()
{
throw new NotImplementedException();
}
public override void TestB()
{
throw new NotImplementedException();
}
public override void TestC()
{
base.TestC();
}
new public void TestD()
{
}
public (bool, bool) TestE()
{
return (true, true);
}
public string TestF<T>(T t)
{
return t.GetType().Name;
}
public string TestG(in string a, ref string aa, out string b, string c = "666")
{
b = "666";
return string.Empty;
}
public string TestH(params string[] d)
{
return string.Empty;
}
}</code></pre>
Copy and paste the parsing methods from section 2.1.4 into the project to parse methods within a class using the code below.
Type type = typeof(MyClass);
MethodInfo[] methods = type.GetMethods(BindingFlags.Public | BindingFlags.Static | BindingFlags.DeclaredOnly | BindingFlags.Instance);
foreach (MethodInfo item in methods)
{
StringBuilder builder = new StringBuilder();
builder.Append(GetVisibility(item) + " ");
builder.Append(item.GetGetMethod(true).IsStatic ? "static " : string.Empty);
builder.Append(IsOver(type, item) + " ");
builder.Append(GetReturn(item) + " ");
builder.Append(GetMethodName(item) + " ");
builder.Append("(" + GetParams(item) + ")");
Console.WriteLine(builder.ToString());
}</code></pre>
No parsing for generic and array types here, nor output of attributes.
Try replacing MyClass with types such as List<> for testing.
Output:
public void TestA ()
public override void TestB ()
public override void TestC ()
public void TestD ()
public (Boolean,Boolean) TestE ()
public String TestF ( T t )
public String TestG (in String a ,ref String aa ,out String b , String c = 666 )
public String TestH (params String[] d )
The complete code has been uploaded to Gitee, click to view Parsing Methods and Parameters.
2.1.5 Constructors
For constructors, there is no return type and no overriding; for the method of getting parameters, we won't elaborate since much of the code is repetitive as in 2.1.4. The code has been uploaded to Gitee for reference Parsing Constructors.
2.1.6 Properties
Normally, writing properties like this is acceptable, but excessive modifiers are meaningless for properties.
public class MyClass
{
public int a { get; set; }
internal int b { get; set; }
protected int c { get; set; }
protected internal int d { get; set; }
private int e { get; set; }
public static float f { get; set; } = 1;
}
PropertyInfo
does not have as rich support for checking modifiers as FieldInfo
.
However, the method to get properties can obtain access modifiers.
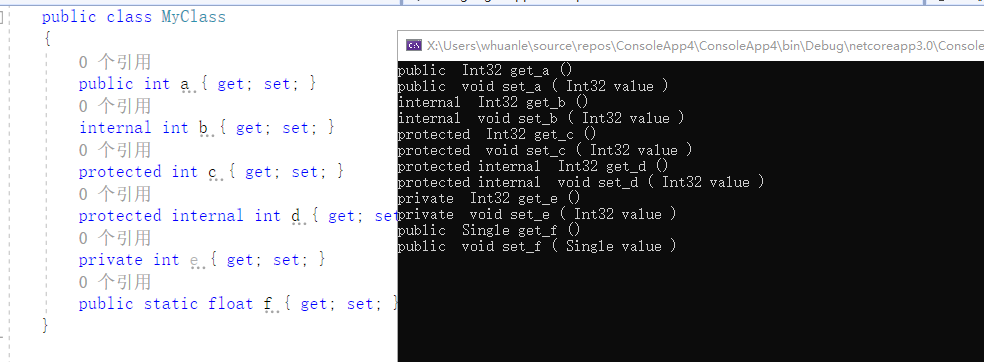
Getting Access Modifiers
Just slightly adjust from getting method access modifiers.
public static string GetVisibility(PropertyInfo property)
{
MethodInfo method = property.GetGetMethod();
return
method.IsPublic ? "public" :
method.IsPrivate ? "private" :
method.IsAssembly ? "internal" :
method.IsFamily ? "protected" :
method.IsFamilyOrAssembly ? "protected internal" :
null;
}
Getting Override Keywords
// virtual override abstract new
public static string IsOver(Type type, PropertyInfo property)
{
MethodInfo method = property.GetGetMethod(true);
// No corresponding information indicates no use of the above keywords
if (!method.IsHideBySig)
return string.Empty;
// Abstract method
if (method.IsAbstract)
return "abstract";
// virtual, override, implementing interface method
if (method.IsVirtual)
{
// Implementing interface method
if (method.IsFinal)
return string.Empty;
// Not overridden, thus virtual
if (method.Equals(method.GetBaseDefinition()))
return "virtual";
else
return "override";
}
// new
else
{
// If the method is defined in the current type, it's just a normal method
if (type == method.DeclaringType)
return string.Empty;
return "new";
}
}</code></pre>
Parsing Property Getters and Setters
// Parse property getters and setters
public static string GetConstructor(PropertyInfo property)
{
string str = "{ ";
if (property.CanRead)
str += "get; ";
if (property.CanWrite)
str += "set; ";
str += "}";
return str;
}
Reflection cannot directly obtain the default value of a property. For details, please refer to https://www.ojit.com/article/3058539.
Above is the test code, which can be viewed on Gitee Parsing Properties.
2.1.7 Events
This section continues to use all the functions for parsing methods from section 2.1.4.
Define delegates and events as follows
public delegate void DeTest();
public abstract class A
{
public abstract event DeTest TestA;
}
public abstract class B : A
{
public virtual event DeTest TestB;
public event DeTest TestC;
}
public class MyClass : B
{
public override event DeTest TestA;
public override event DeTest TestB;
new public event DeTest TestC;
}
Process to Parse Events
Type type = typeof(MyClass);
EventInfo[] events = type.GetEvents(BindingFlags.Public | BindingFlags.Static | BindingFlags.DeclaredOnly | BindingFlags.Instance);
foreach (var item in events)
{
MethodInfo method = item.GetAddMethod();
StringBuilder builder = new StringBuilder();
builder.Append(GetVisibility(method) + " ");
builder.Append(method.IsStatic ? "static " : string.Empty);
builder.Append(IsOver(type, method) + " ");
builder.Append("event ");
builder.Append(item.EventHandlerType.Name + " ");
builder.Append(item.Name + ";");
Console.WriteLine(builder.ToString());
}
The parsing process is quite simple.
2.1.8 Indexer
We define a type and an indexer as follows:
public class MyClass
{
private string[] MyArray;
public MyClass()
{
MyArray = new string[] { "a", "b", "c", "d", "e" };
}
// Search is not processed here
public string this[int index,string search]
{
get
{
return MyArray[index];
}
set
{
MyArray[index] = value;
}
}
}
At compile time, the indexer will generate properties and methods, so when using reflection to obtain properties, the properties generated by the indexer will be included.
The constructor will automatically generate a public string Item { get; set; }
property.
This section uses the code from 2.1.6 to parse properties.
Optimizing the property retrieval method as follows will distinguish the properties in the output type from the constructor.
Type type = typeof(MyClass);
PropertyInfo[] properties = type.GetProperties(BindingFlags.Public | BindingFlags.Static | BindingFlags.NonPublic | BindingFlags.DeclaredOnly | BindingFlags.Instance);
foreach (PropertyInfo item in properties)
{
StringBuilder builder = new StringBuilder();
builder.Append(GetVisibility(item) + " ");
builder.Append(item.GetGetMethod(true).IsStatic ? "static " : string.Empty);
builder.Append(IsOver(type, item) + " ");
builder.Append(item.PropertyType + " ");
if (item.Name == "Item")
{
builder.Append("this[");
ParameterInfo[] paras = item.GetIndexParameters();
int length = paras.Length - 1;
for (int i = 0; i <= length; i++)
{
builder.Append(paras[i].ParameterType.Name + " " + paras[i].Name);
if (i < length)
builder.Append(",");
}
builder.Append("]");
}
else
{
builder.Append(item.Name + " ");
builder.Append(GetConstructor(item));
}
Console.WriteLine(builder.ToString());
}</code></pre>
2.1.9 Getting Attributes
Types, methods, properties, fields, etc., can be modified by attributes. We need to retrieve attributes through reflection and then restore the values set by the programmer when writing code.
The code is as follows:
///
/// Parses attributes of types, methods, properties, fields, etc.
///
///
///
public static string[] GetAttrs(IList attrs)
{
List attrResult = new List(); ;
foreach (var item in attrs)
{
Type attrType = item.GetType();
string str = "[";
str += item.AttributeType.Name;
// Values in the constructor
IList customs = item.ConstructorArguments;
// Values of properties
IList arguments = item.NamedArguments;
// No values
if (customs.Count == 0 && arguments.Count == 0)
{
attrResult.Add(str + "]");
continue;
}
str += "(";
if (customs.Count != 0)
{
str += string.Join(",", customs.ToArray());
}
if (customs.Count != 0 && arguments.Count != 0)
str += ",";
if (arguments.Count != 0)
{
str += string.Join(",", arguments.ToArray());
}
str += ")";
attrResult.Add(str);
}
return attrResult.ToArray();
}</code></pre>
Invocation:
Type type = typeof(List<>);
string[] list = GetAttrs(type.GetCustomAttributesData());
foreach (var item in list)
{
Console.WriteLine(item);
}
During invocation, change Type to MethodInfo, etc.
Output:
[SerializableAttribute]
[DebuggerDisplayAttribute("Count = {Count}")
[NullableAttribute((Byte)0)
[DebuggerTypeProxyAttribute(typeof(System.Collections.Generic.ICollectionDebugView`1))
[NullableContextAttribute((Byte)1)
[DefaultMemberAttribute("Item")
[TypeForwardedFromAttribute("mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089")
2.2 Delegate
Here we use the code from 2.1.4 to parse methods.
A delegate may contain many methods, among which there is an invoke
method that corresponds to various information defined for the delegate.
///
/// Parses delegates, including delegates in nested types
///
///
///
public static string GetDelegateInfo(Type type)
{
if (!type.IsSubclassOf(typeof(Delegate)))
return null;
string str = "";
MethodInfo method = type.GetMethod("Invoke");
if (type.IsNested)
str += GetVisibility(method);
else
str += (type.IsPublic ? "public" : "internal") + " ";
str += type.IsSealed && type.IsAbstract ? "static " : string.Empty;
str += "delegate ";
str += GetReturn(method) + " ";
str += type.Name;
str += "(";
str += GetParams(method);
str += ")";
return str;
}</code></pre>
2.3 Interface
Having parsed classes, abstract classes, and delegates, we can use the same approach to parse interfaces and then analyze the properties and methods of the interface.
No further elaboration will be provided here.
2.4 Nullable Types
To determine whether a type is a nullable type, you can first check whether it is a generic type.
Both nullable types and generic methods can be judged using the IsGenericType
property.
The GetGenericTypeDefinition
method can retrieve the unbound parameter version of the generic type.
Finally, check if the type is typeof(Nullable<>)
to complete the overall analysis.
///
/// Gets the name of the nullable type
///
///
public static string GetAbleNullName(Type type)
{
if (!type.IsGenericType)
return type.Name;
if (type.GetGenericTypeDefinition() == typeof(Nullable<>))
{
Type nullType = type.GetGenericArguments().FirstOrDefault();
return nullType.Name + "?";
}
return type.Name;
}</code></pre>
文章评论