- RuntimeInformation and Environment
- Fetching Information
- Reflection to Fetch Information
- Fetching a Property's Value and Alias
- Reflection to Fetch Information
- Usage
- Summary
The author’s nine articles on the reflection system have concluded, but the author will continue to update the applications of reflection in daily coding. This article mainly covers retrieving runtime environment information in .NET Core and using reflection to handle data more conveniently. The content includes: RuntimeInformation, Environment, reflection, attributes, etc. The code download link is here.
Example of retrieval:
The links to the author's nine reflection series articles are as follows:
C# Reflection and Attributes (1): Basics of Reflection
C# Reflection and Attributes (2): Exploring Reflection
C# Reflection and Attributes (3): Reflection Type Members
C# Reflection and Attributes (4): Instantiating Types
C# Reflection and Attributes (5): Type Member Operations
C# Reflection and Attributes (6): Implementing ASP.NET Core Dependency Injection Web
C# Reflection and Attributes (7): Custom Attributes and Their Applications
C# Reflection and Attributes (8): Comprehensive Examples of Reflection Operations
C# Reflection and Attributes (9): Comprehensive Analysis of Reflection Examples
RuntimeInformation and Environment
The RuntimeInformation class provides information about the .NET runtime installation. It primarily retrieves the platform and version, with fewer APIs. Documentation can be found at here. The Environment class provides information about the current environment and platform as well as methods to manipulate them. It has more APIs. Documentation can be found at here.
The above two classes already have documentation links, so they will not be elaborated further. It is important to note that there are differences between Windows and Linux, and some APIs are not cross-platform. Additionally, .NET Core has significantly fewer APIs for retrieving system resource information compared to .NET Framework. .NET Core does not have APIs to get system CPU and memory usage; however, it is possible to obtain the current process's CPU and memory usage. More information can be found on StackOverflow at this link.
Fetching Information
The following retrieves memory used by the process and used CPU time. CPU time is not as intuitive as the directly acquired usage percentage. The formula for CPU time is as follows:
CPU Time = Number of clock cycles needed to execute the program * Clock cycle time
Since CPUs have multiple cores and threads, calculating based on how long the process has run may not be accurate. Moreover, processes can encounter sleep and context switching situations. Thus, a program that has been running for several hours may only have a CPU time of several minutes. For more details on CPU performance calculation methods, please refer to this article. For details on CPU utilization calculations in Linux, please check this article.
The code we use in C# is as follows:
[Display(Name = "Running Information")]
public class ApplicationRunInfo
{
private double _UsedMem;
private double _UsedCPUTime;
public ApplicationRunInfo()
{
var proc = Process.GetCurrentProcess();
var mem = proc.WorkingSet64;
var cpu = proc.TotalProcessorTime;
_UsedMem = mem / 1024.0;
_UsedCPUTime = cpu.TotalMilliseconds;
}
[Display(Name = "Physical memory used by the process (kb)")]
public double UsedMem { get { return _UsedMem; } }
[Display(Name = "CPU time consumed by the process (ms)")]
public double UsedCPUTime { get { return _UsedCPUTime; } }
}
There are only two properties here. We use the Display attribute to mark the meaning of this property for easier information retrieval through reflection.
Additionally, there are two classes to fetch different types of information as follows:
[Display(Name = "System Platform")]
public class SystemPlatformInfo
{
[Display(Name = "Runtime Framework")]
public string FrameworkDescription { get { return RuntimeInformation.FrameworkDescription; } }
[Display(Name = "Operating System")]
public string OSDescription { get { return RuntimeInformation.OSDescription; } }
[Display(Name = "Operating System Version")]
public string OSVersion { get { return Environment.OSVersion.ToString(); } }
[Display(Name = "Platform Architecture")]
public string OSArchitecture { get { return RuntimeInformation.OSArchitecture.ToString(); } }
}
[Display(Name = "Runtime Environment")]
public class SystemRunEvnInfo
{
[Display(Name = "Machine Name")]
public string MachineName { get { return Environment.MachineName; } }
[Display(Name = "User Domain Name")]
public string UserDomainName { get { return Environment.UserDomainName; } }
[Display(Name = "Logical Disks")]
public string GetLogicalDrives { get { return string.Join(", ", Environment.GetLogicalDrives()); } }
[Display(Name = "System Directory")]
public string SystemDirectory { get { return Environment.SystemDirectory; } }
[Display(Name = "System Uptime (milliseconds)")]
public int TickCount { get { return Environment.TickCount; } }
[Display(Name = "Running in Interactive Mode")]
public bool UserInteractive { get { return Environment.UserInteractive; } }
[Display(Name = "Current User Name")]
public string UserName { get { return Environment.UserName; } }
[Display(Name = "Web Program Core Framework Version")]
public string Version { get { return Environment.Version.ToString(); } }
// Not valid for Linux
[Display(Name = "System Drive")]
public string SystemDrive { get { return Environment.ExpandEnvironmentVariables("%SystemDrive%"); } }
// Not valid for Linux
[Display(Name = "System Root")]
public string SystemRoot { get { return Environment.ExpandEnvironmentVariables("%SystemRoot%"); } }
}
You may wonder why this is written in such an odd way instead of as methods. Just be patient and keep reading~
Reflection to Fetch Information
We will define a static class as the entry point for fetching various information.
public static class EnvironmentInfo
{
}
}
Fetching Property Values
This method retrieves the property values of the classes mentioned above using reflection.
///
/// Get the value of a property
///
///
/// Instance
///
private static object GetPropertyInfoValue(PropertyInfo info, object obj)
{
return info.GetValue(obj);
}
Reflection to Fetch Attribute Values
We use the attribute [Display(Name = "Current User Name")]
to store the alias. We need to retrieve the Name property value of the Display attribute through reflection.
///
/// Get the value of the Name property of [Display] attribute
///
///
///
private static string GetDisplayNameValue(IList attrs)
{
var argument = attrs.FirstOrDefault(x => x.AttributeType.Name == nameof(DisplayAttribute)).NamedArguments;
return argument.FirstOrDefault(x => x.MemberName == nameof(DisplayAttribute.Name)).TypedValue.Value.ToString();
}
Fetching a Property's Value and Alias
We use this way to set and retrieve information:
[Display(Name = "Operating System")]
public string OSDescription { get { return RuntimeInformation.OSDescription; } }
Thus, we want to retrieve all property values and their attribute values for a type.
///
/// Get the values and names of a certain type
///
///
///
///
private static (string, List>) GetValues(TInfo info)
{
List> list = new List>();
Type type = info.GetType();
PropertyInfo[] pros = type.GetProperties();
foreach (var item in pros)
{
var name = GetDisplayNameValue(item.GetCustomAttributesData());
var value = GetPropertyInfoValue(item, info);
list.Add(new KeyValuePair(name, value));
}
return
(GetDisplayNameValue(info.GetType().GetCustomAttributesData()),
list);
}
Reflection to Get Information
After defining the above utility method, let's set different methods to obtain different information.
///
/// Get application run resource information
///
///
public static (string, List>) GetApplicationRunInfo()
{
ApplicationRunInfo info = new ApplicationRunInfo();
return GetValues(info);
}
/// <summary>
/// Get system platform information
/// </summary>
/// <returns></returns>
public static (string, List<KeyValuePair<string, object>>) GetSystemPlatformInfo()
{
SystemPlatformInfo info = new SystemPlatformInfo();
return GetValues(info);
}
/// <summary>
/// Get system running environment information
/// </summary>
/// <returns></returns>
public static (string, List<KeyValuePair<string, object>>) GetSystemRunEvnInfo()
{
SystemRunEvnInfo info = new SystemRunEvnInfo();
return GetValues(info);
}</code></pre>
There is also a method to obtain environment variables that does not require using the above type-property manipulation and can be directly encapsulated in the method.
///
/// Get all system environment variables
///
///
public static (string, List>) GetEnvironmentVariables()
{
List> list = new List>();
IDictionary environmentVariables = Environment.GetEnvironmentVariables();
foreach (DictionaryEntry de in environmentVariables)
{
list.Add(new KeyValuePair(de.Key.ToString(), de.Value));
}
return ("System Environment Variables", list);
}
Usage
In Program, writing these will output all the information.
static void Main(string[] args)
{
var a = EnvironmentInfo.GetApplicationRunInfo();
var b = EnvironmentInfo.GetSystemPlatformInfo();
var c = EnvironmentInfo.GetSystemRunEvnInfo();
var d = EnvironmentInfo.GetEnvironmentVariables();
ConsoleInfo(a.Item1, a.Item2);
ConsoleInfo(b.Item1, b.Item2);
ConsoleInfo(c.Item1, c.Item2);
ConsoleInfo(d.Item1, d.Item2);
Console.ReadKey();
}
public static void ConsoleInfo(string title, List> list)
{
Console.WriteLine("\n***********" + title + "***********");
foreach (var item in list)
{
Console.WriteLine(item.Key + ":" + item.Value);
}
}
Displayed in Linux
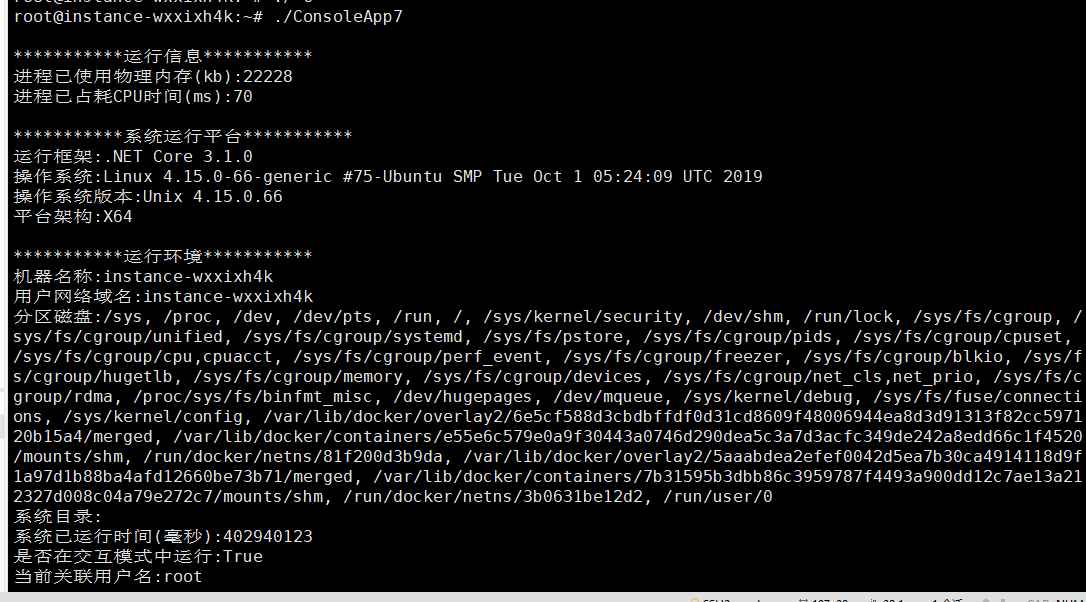
Conclusion
I have used class-property for obtaining functionality above, so that we do not need to write many methods to call and get environment information, as properties are the data. This is convenient for serialization and reflection.
At the same time, if we want to extend the information items, we can directly add them, and reflection will obtain all of them.
Additionally, there is a Display attribute specifically used for displaying information items. This setup allows for flexible aliasing of properties, facilitating the display of information and explanations.
The author will continue to bring more examples of using reflection, incorporating them into daily needs.
文章评论