There are two toolkits for writing GUI in Java, namely AWT and Swing.
Swing is an extension of AWT, featuring richer components and methods compared to AWT.
Both AWT and Swing can be used across platforms; however, AWT UI styles may vary with different system platforms, whereas Swing maintains a consistent style across all platforms once designed.
import java.awt.*;
import javax.swing.*;
An AWT Example
Below is a window example.
import java.awt.*;
public class MyFrame extends Frame {
public MyFrame(){
super("Testing");
setSize(400,200);
setVisible(true);
}
public static void main(String args[]){
new MyFrame();
}
}
To create a window, you need to extend Frame
and inherit some methods from Frame.
In Frame:
- The
super
method sets the window title; setSize
sets the window size,(width,height)
setVisible
determines whether the window is displayed; true shows it, false hides it.
setLayout(new FlowLayout());
is used to set the window layout;
add(component);
is used to add components to the window, such as buttons.
Let’s add a component to the window.
import java.awt.*;
public class MyFrame extends Frame {
public MyFrame(){
super("Testing");
setLayout(new FlowLayout());
Button btn=new Button("Button");
Font f=new Font("SimSun",Font.BOLD,28);
btn.setFont(f);
add(btn);
setSize(400,200);
setVisible(true);
}</code></pre>
Start the window in the main method.
public static void main(String args[]){
new MyFrame();
}
Effect:
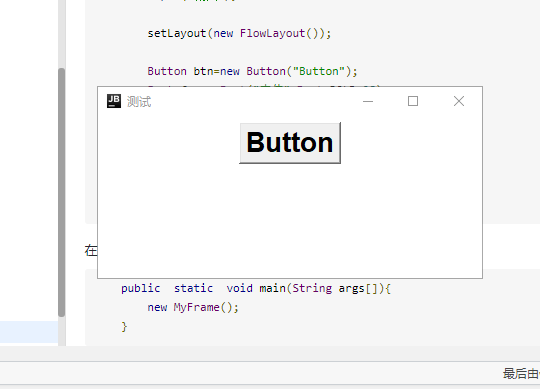
Events
In Java, events are described in three aspects:
Event source: The object that triggers the event
Event handling: Delegation event handling model
Event listener: Responsible for handling the event
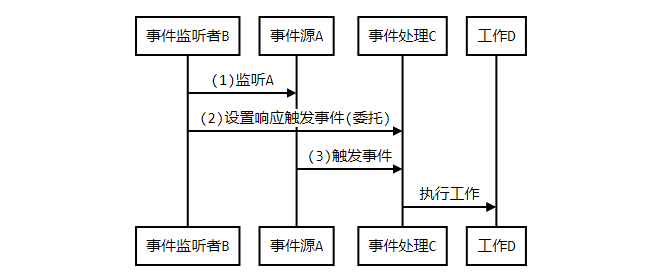
First, B listens to A, sets the listening content, and sets the response content.
Once A's state changes, it triggers C;
C performs D.
A mother tells her son, "If you finish your homework, I will ask dad to peel an apple for you."
Mother: Event monitor
Son: Event source
Action: Finishing homework
Event: Dad peels an apple for the son
The mother monitors her son to check if he has finished his homework. Once the homework is completed, it triggers the event: peeling the apple;
When the son finishes his homework, the state changes, triggering the event; and the event starts executing.
In simple terms, B tells A that if A does something, then C will be executed.
Implementing Event Flow
We continue using the window above to change the window background color when the button is clicked.
Implementing an Event
Import:
import java.awt.event.ActionListener;
Implement the interface:
class Test implements ActionListener{
public void actionPerformed(ActionEvent actionEvent) {
System.out.println("Executing work");
}
Registering the Event to the Control
package com.company;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MyFrame extends Frame implements ActionListener {
public MyFrame(){
super("Testing");
setLayout(new FlowLayout());
Button btn=new Button("Button");
Font f=new Font("SimSun",Font.BOLD,28);
btn.setFont(f);
btn.addActionListener(this::actionPerformed);
add(btn);
setSize(400,200);
setVisible(true);
}
public void actionPerformed(ActionEvent actionEvent) {
System.out.println("Executing work");
setBackground(Color.BLUE);
}
}
The button has an event listener addActionListener
, where we bind the work to be executed actionPerformed
.
When the condition is met, this work will be triggered.
I have no idea what I’m talking about.
In C#, events aren’t that complicated and don’t need this much unnecessary detail...
Additionally, actionEvent
has two commonly used methods:
actionEvent.getActionCommand(); // Get object name
actionEvent.getSource(); // Get source object
Layouts
In AWT, there are mainly 6 types of layout.
- FlowLayout: A flow layout
The default layout arranges components from top to bottom, left to right.
- BorderLayout: A border layout
Automatically docks the components to the edges of the window and resizes with the window changes.
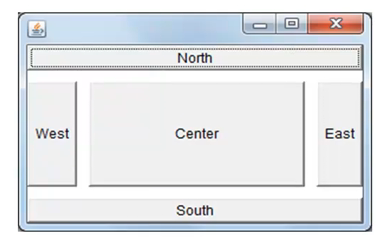
- GridLayout: A grid layout
Divides the container into several rows and columns.
- CardLayout: A card layout
Each card is a component like a playing card, multiple components stacked, and only one is visible at a time.
- GridBagLayout: A grid bag layout
- null: Uses coordinate positioning layout
Create a window and set a flow layout:
public class MyFrame extends Frame {
public MyFrame(){
super("Testing");
setLayout(new FlowLayout());
}
}
In a frame that inherits Frame, you can set the layout using the setLayout
method.
Component Methods
The following is a common relationship inheritance diagram of GUI controls.
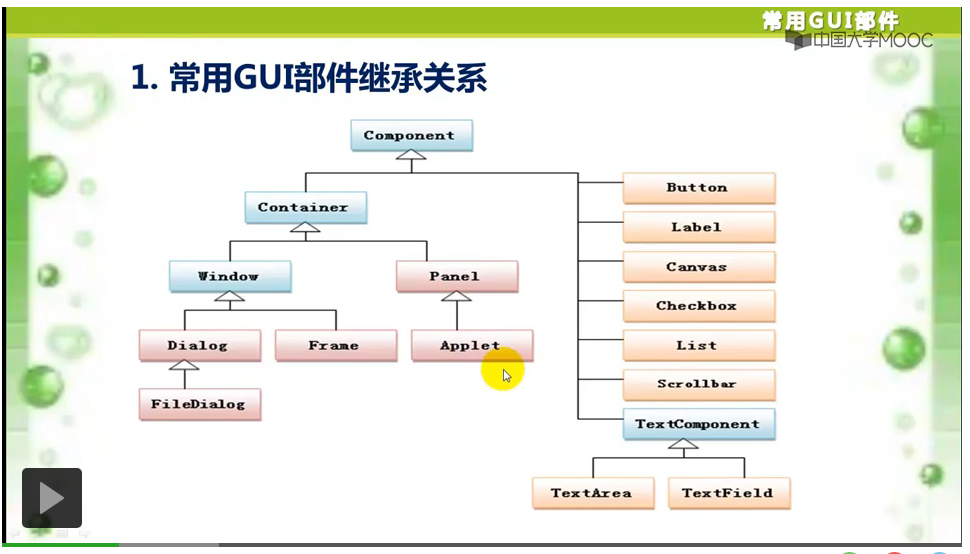
Components are divided into containers (Container) and controls.
Containers are further divided into windows (Window) and panels (Panel).
The Component class is the parent class of all components, and the common methods for the Component class are as follows:
Component class (abstract class) main methods
Color getBackground() : Get the component's background color
Font getFont() : Get the component's display font
Graphics getGraphics(): Get the Graphics object of the component
void setBackground(Color c) : Set the component's background
void setEnabled(boolean b) : Whether to enable the component's functionality
void setFont(Font f) : Set the component's display font
void setSize(int width,int height) : Set the component size
void setVisible(boolean b) : Set whether the component is visible
void setForeground(Color c) : Set the component's foreground color
Toolkit getToolkit() : Get the toolkit for graphical components
void requestFocus() : Request focus for the component
void add(PopupMenu popup) : Add a popup menu to the component
Common Controls
Text
The text field (TextField) can only display one line of text, while the text area (TextArea) can display and edit multi-line text.
The constructors for the text field are as follows:
TextField(): Constructs a single-line text input box.
TextField(int): Constructs a single-line text input box with specified length.
TextField(String): Constructs a single-line text input box with specified initial content.
TextField(String, int): Constructs a single-line text input box with specified length and initial content.
The constructors for the text area are as follows:
TextArea(): Constructs a text area.
TextArea(int, int): Constructs a text area with specified length and width.
TextArea(String): Constructs a text area displaying specified text.
TextArea(String, int, int): Constructs a text area with specified length, width, and default value.
The commonly used methods for text controls are:
void setEchoChar('*') : Set the echo character
String getText() : Get the data in the input box
void setText(String s) : Write data to the input box
boolean isEditable() : Check if the input box is editable.
void select(int start, int end) : Select the text specified by the start and end positions.
void selectAll() : Select all text.
The text area (TextArea) also has the following two commonly used methods:
append(String s) : Append the string to the end of the text area
insert(String s, int index) : Insert the string at the specified position in the text area
Text fields have two common events:
ActionEvent
Triggered when the Enter key is pressed in the text field.
Registration: addActionListener()
Interface: ActionListener
Method: public void actionPerformed(ActionEvent e)
TextEvent
For the text input component's data modification operations (add, modify, delete).
Registration: addTextListener()
Interface: TextListener
Method: public void textValueChanged(TextEvent e)
文章评论