C/C++、C#、JAVA(三):字符串操作
Table of Contents
C/C++、C#、JAVA(三):字符串操作
- Defining Strings
- Catching Input and Output
- Value Comparison
String Operations - String Search
- String Extraction, Insertion, Deletion, Replacement
Defining Strings
C
In C, there is no string object; strings are stored using char []
.
char a[] = "abcdef";
char c[6] = {'a', 'b', 'c', 'd', 'e', '\0'};
char* b = a;
In C, strings must end with \0
.
When defining an array, an extra position must be provided to store \0
.
C++
In the C++ standard library, strings are represented using string. String objects can be concatenated directly.
#include <iostream>
#include <string>
using namespace std;
int main ()
{
string str1 = "Hello";
string str2 = "World";
string str3;
int len ;
// Copy str1 to str3
str3 = str1;
cout << "str3 : " << str3 << endl;
// Concatenate str1 and str2
str3 = str1 + str2;
cout << "str1 + str2 : " << str3 << endl;
// Total length of str3 after concatenation
len = str3.size();
cout << "str3.size() : " << len << endl;
return 0;
}
// Example from https://www.runoob.com/cplusplus/cpp-strings.html
C#
In C#, strings can be declared directly.
string a = "abcdef";
JAVA
In JAVA, strings are declared using String.
String a = "1234";
String b = new String("1234");
Catching Input and Output
In C, there are several functions to receive user input from the keyboard:
scanf()
can input various data types, whileprintf()
can output various data types;getchar()
can obtain a single character, andputchar()
outputs a single character;gets()
: obtains a line of data and treats it as a string;puts()
outputs a line of string.
In C++, you can use cin to obtain input strings;
In C#, you can use Console.ReadLine()
to get a string, and Console.ReadKey()
to obtain the pressed key.
In JAVA, you can use the System.in
class or Scanner
class and BufferedReader
class to input strings, and use System.out
class to output strings.
When I was learning JAVA, I found the way to obtain input complicated after searching on Baidu. The following examples show the process of obtaining input in various languages.
Below are the core syntax of each language, please remember:
C Language Version
char a[10] = "\0";
gets_s(a); // In VC, gets is replaced by the safer gets_s
puts(a);
scanf("%s", a); // Both with and without & work
printf("%s",a);</code></pre>
C++ Version
string a;
cin >> a;
cout << a << endl;
C# Version
string a;
a = Console.ReadLine();
JAVA Version
String a;
Scanner input = new Scanner(System.in);
a = input.nextLine();
System.out.println(a);
input.close();
I find JAVA to be a hassle to use.
C, C++, and C# methods are easy to learn at a glance, while JAVA requires importing import java.util.Scanner;
.
Value Comparison
C/C++
Let's write a C++ program.
string str1 = "1";
string str2 = "1";
cout << (str1 == str2) << endl;
char str3[] = "1";
char str4[] = "1";
cout << (str1 == str2) << endl;
char str5[] = { '1','\0' };
char str6[] = { '1','\0' };
cout << (str5 == str6) << endl;
cout << (str1 == str3) << endl;</code></pre>
The result is 1,1,0,1
.
str1, str2, str3, and str4 are equal to each other as they all refer to the constant 1.
C#
In C#, string is a reference type.
==
compares values for value types; for reference types, it compares whether the reference addresses are equal.
Since the string type overrides the operator, ==
for strings compares whether the strings are the same.
string a = "abcd";
string b = "abcd";
Console.WriteLine(a==b);
The result is true.
The string comparison in C# is very rich and offers strong support for character globalization.
We will not discuss this further.
JAVA
In JAVA, we find int -> Integer, double -> Double, etc., but there is only one String.
In JAVA, String does not override the ==
operator, so it still compares reference addresses.
To compare whether strings are the same, you can use equals
.
String a = "1234";
String b = new String("1234");
System.out.println(a == b); // false
String c = "1234";
System.out.println(a == c); // true
System.out.println(a.equals(b)); // true
String d = "abc";
String e = "ABC";
System.out.println(d.equalsIgnoreCase(e)); // true, ignoring case</code></pre>
String Operations
String Search
C
In C, the strchr
function is used to locate the first occurrence of a specified character in a string (stops at \0
). It returns a pointer.
We can convert the pointer to an index position as follows:
char a[] = "abcdefghijklmnopq";
char* p = strchr(a, 'c');
// If not found, it returns NULL, please check
printf("%d", p - a);
memchr
works similarly to strchr
, but does not stop when encountering \0
.
char a[] = { 'a','\0','b','c','d' };
char* p = strchr(a, 'c');
if (p == NULL)
printf("Not found\n");
else
printf("Index position is: %d", p - a + 1);
// memchr returns void*, need to cast to char*
p = (char *)memchr(a, 'c', sizeof(a));
if (p == NULL)
printf("Not found\n");
else
printf("Index position is: %d", p - a + 1);</code></pre>
The strstr()
method can search for the position of a string.
char a[] = "Hello world!\0";
char* p = strstr(a, "world");
if (p == NULL)
printf("Not found\n");
else
printf("Index position is: %d", p - a + 1);
C++
In C++, there are various ways to find characters, such as find()
and rfind()
, which will not be discussed in detail here.
find()
and rfind()
can search for characters and strings.
Examples are as follows:
string a = "my name is 痴者工良,hello,any boy~";
cout << a.find('n') << endl;
cout << a.find("name") << endl;
cout << a.find("name", 8) << endl; // Search starting from index 8
cout << a.find("namez") << endl;
// In the above two outputs, not found will lead to outputting incorrect values
// This can be solved as follows
// If not found, it will output -1
string::size_type index = a.find("namez");
cout << (int)index << endl;</code></pre>
The rfind()
function works the same as find()
, but rfind()
is a reverse search.
string a = "my name is 痴者工良,hello,any boy~";
cout << a.rfind('n') << endl;
cout << a.rfind("name") << endl;
cout << a.rfind("name", 8) << endl; // Search starting from index 8
cout << a.rfind("namez") << endl;
// In the above two outputs, not found will lead to outputting incorrect values
// This can be solved as follows
// If not found, it will output -1
string::size_type index = a.find("namez");
cout << (int)index << endl;</code></pre>
The rfind()
function checks backward and returns the index position if successful.
The find_first_of()
and find_last_of()
functions can be used to find the first and last occurrence of a character or string.
For reference, see http://c.biancheng.net/view/1453.html
C#
C# has many search methods which are easy to use.
For specifics, refer to another article by the author https://www.cnblogs.com/whuanle/p/11967014.html#4-systemstring-字符串.
StartsWith()
and EndsWith()
can use StringComparison to compare styles and CultureInfo to control culture-related rules.
StartsWith()
: checks if the string starts with the matching string.
EndsWith()
: checks if the string ends with the matching string.
Contains()
: checks if the matching string exists at any position in the string.
IndexOf
: finds the first occurrence of the string or character index position, where a return value of -1
indicates no match found.
Usage example:
string a = "痴者工良(高级程序员劝退师)";
Console.WriteLine(a.StartsWith("高级"));
Console.WriteLine(a.StartsWith("高级",StringComparison.CurrentCulture));
Console.WriteLine(a.StartsWith("高级",true, CultureInfo.CurrentCulture));
Console.WriteLine(a.StartsWith("痴者",StringComparison.CurrentCulture));
Console.WriteLine(a.EndsWith("劝退师)",true, CultureInfo.CurrentCulture));
Console.WriteLine(a.IndexOf("高级",StringComparison.CurrentCulture));
Output:
False
False
False
True
True
5
JAVA
In JAVA, the string's indexOf()
method returns the position of the first occurrence of a string, while lastIndexOf()
returns the position of the last occurrence.
startsWith
checks if a string starts with a particular string.
regionMatches
is used to handle globalization issues.
Examples are omitted; please refer to C# examples.
String Extraction, Insertion, Deletion, Replacement
I won't elaborate on C#, please refer to another article by the author:
https://www.cnblogs.com/whuanle/p/11967014.html#42-字符串提取、插入、删除、替换
C
The strncpy()
function can copy multiple bytes from a source string to another string. It is suitable for truncating strings and includes \0
. strncpy()
works like strcpy()
, except it does not include \0
.
strcpy
places one string into another string, overwriting it.
char a[] = "my name is 痴者工良,hello,any boy~\0";
char b[4] = { '1','1','1','\0' };
// b <- a
strncpy(b, a, 3); // Truncate to the first three characters
printf("%s\n", b);
char aa[] = "my name is 痴者工良,hello,any boy~\0";
char bb[4] = { '1','1','1','\0' };
strncpy(bb, aa + 5, 3); // Start from the fifth character and take three characters
printf("%s\n", bb);
char c[] = "123456";
char d[] = "a";
// c <- d
strcpy(c, d);
puts(d);
return 0;</code></pre>
Refer to https://www.cnblogs.com/jixiaohua/p/11330096.html for more information.
In C, there are no official support functions for inserting, deleting, or replacing strings, or I’m not aware of any. 😜
。
Earlier, we learned about the string search function strstr()
in C language, which we can use to obtain the start and end positions of a string for subsequent operations.
C++ and JAVA
C++, C#, and JAVA all have the sub
method, and their usage is quite similar.
string a = "my name is whuanle,hello,any boy~";
cout << a.substr(0,5) << endl; // Start at index 0, extract 5 characters
cout << a.substr(5, 5) << endl; // Start at index 5, extract 5 characters
String a = "my name is whuanle,hello,any boy~";
System.out.println(a.substring(0,5));
System.out.println(a.substring(2,7));
The sub
method in C++ and C# has similar parameters, where the left parameter represents the starting index and the right parameter represents the number of characters to extract.
In JAVA, the left parameter represents the starting index, while the right parameter represents the ending index.
Additionally, there are methods for inserting, deleting, and replacing... I feel like I'm going crazy...
When I set the flag to write comparison articles on these four languages, I was probably just feeling too full at the time.
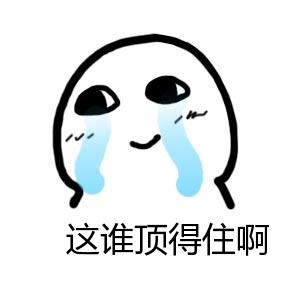
With limited energy, I need to go to sleep early,
For C++ string operations, see ↓
http://c.biancheng.net/view/1449.html
For JAVA string operations, see ↓
https://www.cnblogs.com/freeabyss/archive/2013/05/15/3187057.html
文章评论